Welcome!
This site is still under construction; I'm hoping to get my own build system working.
I plan to use this site to write about technology, acessibility, and design, and to share some art I make.
Meanwhile, here's a quick tutorial about how to add an auto-switching dark mode to your website, without JavaScript!
Media queries let you enable or disable pieces of CSS depending on certain information. We want the
prefers-color-scheme
query, which says whether the device is in light or dark mode. For example, this
code will use a different set of colors for light and dark mode:
@media (prefers-color-scheme: light) {
body {
color: black;
background-color: white;
}
}
@media (prefers-color-scheme: dark) {
body {
color: lightgray;
background-color: black;
}
}
However, that means that you need two copies of your CSS, one for light mode and one for dark mode. We can fix that problem with variables, which let you define a value once, and refer to it many times. This code defines some variables, and then uses them to set the colors:
:root {
--text: black;
--bg: white;
}
body {
color: var(--text);
background-color: var(--bg);
}
If you use a media query to set the variables, then you only need to have one version of your CSS, and it will automatically update based on the device's setting. Here's the finished code:
@media (prefers-color-scheme: light) {
:root {
--text: black;
--bg: white;
}
}
@media (prefers-color-scheme: dark) {
:root {
--text: lightgray;
--bg: black;
}
}
body {
color: var(--text);
background-color: var(--bg);
}
It's worth noting that variables are also pretty useful on their own, since they let you easily swap out colors and other values. They can make your code easier to read, too, since you're giving a name to every color.
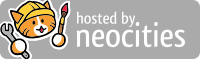